Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 138476
- 노마드코더
- H-index
- Outlet
- usesetrecoilstate
- Typescript
- Helmet
- React
- 티스토리챌린지
- 프로그래머스
- 42747
- Recoil
- 오블완
- programmers
- useoutletcontext
- 귤 고르기
- userecoilvalue
Archives
- Today
- Total
오늘도 코딩하나
[Typescript] 타입스크립트로 블록체인 만들기_(4) 본문
https://nomadcoders.co/typescript-for-beginners
타입스크립트로 블록체인 만들기 – 노마드 코더 Nomad Coders
Typescript for Beginners
nomadcoders.co
강의 내용
#4.0 ~ #4.1
○ Class
class Player {
constructor(
private firstName:string,
private lastName:string,
public nickname:string
) { }
}
const tistory = new Player("코딩하나", "오늘도", "tistory");
// Property 'firstName' is private and only accessible within class 'Player'.
tistory.firstName
- private
: 인스턴스 밖에서 접근할 수 없고, 다른 자식 클래스에서도 접근할 수 없음 - tistory.nickname은 public이라 정상이지만, tistory.firstName은 private이라 오류 발생함.
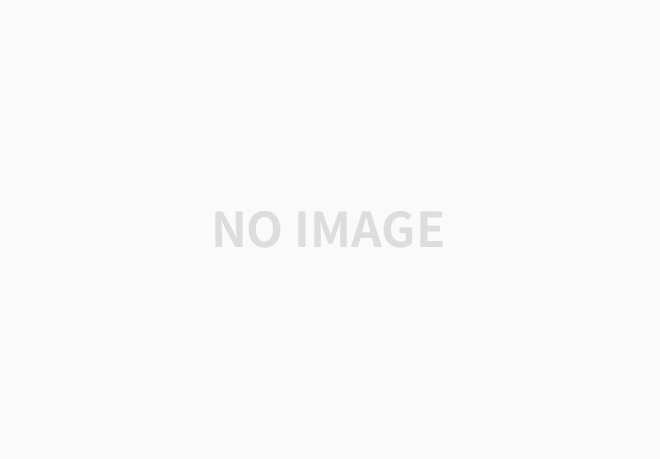
- Javascript로 컴파일되면서 private, public은 사라진 걸 확인해볼 수 있다.
- Javascript에서는 private이나 public같은 보호를 제공하지 않아 아무 문제없이 작동한다.
- 하지만 Typescript는 private, public을 지원하기에 Javascript와 달리 컴파일되지 않는다.
○ Abstract class (추상 클래스)
※ Abstract class : 다른 클래스가 상속받을 수 있는 클래스, 직접적으로 인스턴스를 만들지는 못한다.
// Abstract class
abstract class User {
constructor (
private firstName:string,
private lastName:string,
private nickname:string
) {}
// Abstract method
abstract getNickName():void
getFullName() {
return `${this.firstName} ${this.lastName}`
}
}
class Player extends User {
getNickName() {
// Property 'nickname' is private and only accessible within class 'User'.
console.log(this.nickname)
}
}
const tistory = new Player("오늘도", "코딩", "하나");
tistory.getNickName();
✅ 추상 클래스
- 추상클래스는 직접적으로 인스턴스를 만들지 못한다. ( 대신 상속은 가능! )
- 추상클래스 안에 추상메소드를 만들 수 있다.
✅ 추상 메소드
- 추상 메소드는 구현이 되어 있지 않은 메소드이며, 메소드의 call signature만 제공한다.
- 추상메소드를 상속받은 클래스에서 추상 메소드를 구현한다.
- property를 private로 만든다면, 클래스를 상속하였더라도 그 property에 접근할 수 없고, 오류가 발생한다.
▶ 필드가 외부로부터 보호되지만 다른 자식 클래스에서는 사용되기를 원한다면 protected를 쓰면 된다!
→ nickname 파라미터를 protected로 변경하면 Player클래스에서 this.nickname 사용가능!
✅ private vs public vs protected
- 기본적으로는 public, 외부의 모든 곳에서 사용가능하게 만들려면
- private : 상속 불가, 클래스 내에서만 사용가능
- protected : 상속하는 모든 클래스에서 사용 가능하도록 만들고 싶다면
○ Practice_dictionary
// object의 type을 선언해야할 때 쓸 수 있고
// 이 object는 제한된 양의 property만을 가질 수 있고
// property의 이름은 모르지만 타입만을 알 때 이걸 쓴다.
type Words = {
[key:string]: string
}
class Dict {
// property가 constructor부터 바로 초기화되지 않는다.
private words:Words
constructor() {
// 수동으로 초기화
this.words = {}
}
// 클래스를 타입처럼 사용할 수 있다.
// 파라미터가 클래스의 인스턴스이기를 원하면 이렇게 쓸 수 있다
add(word:Word) {
if(this.words[word.term] === undefined) {
this.words[word.term] = word.def;
}
}
def(term:string) {
return this.words[term]
}
}
class Word {
constructor (
public term:string,
public def :string
) {}
}
const kimchi = new Word("kimchi", "한국의 음식");
const dict = new Dict()
dict.add(kimchi)
dict.def("kimchi")
'Typescript > Lecture' 카테고리의 다른 글
[Typescript] 타입스크립트로 블록체인 만들기_Dictionary (1) | 2025.01.09 |
---|---|
[Typescript] 타입스크립트로 블록체인 만들기_(5) (0) | 2025.01.09 |
[Typescript] 타입스크립트로 블록체인 만들기_(3) (1) | 2025.01.07 |
[Typescript] 타입스크립트로 블록체인 만들기_(2) (0) | 2025.01.06 |
[Typescript] 타입스크립트로 블록체인 만들기_(1) (1) | 2025.01.06 |